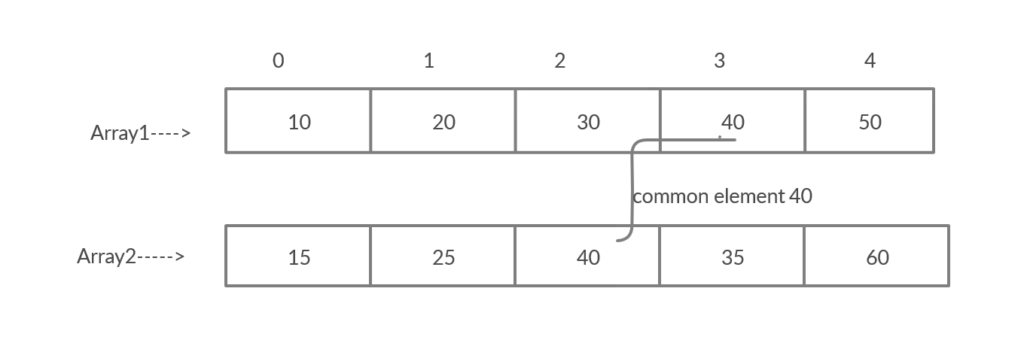
program-to-find-common-elements-between-two-arrays. The above diagram depicts two arrays: array1 and array 2. Both arrays consist of elements. So in this program, our aim is to compare these two array elements and thus find the common element that exits. If there is a common element, then display the common element, If not, display there are no common elements.
Example 1: Taking Static Input
Algorithm/Program Explanation
- start
- define and initialize two array
- int array1[]={data};
- int array2[]={data};
- define 2 for loops
- for(int i=0;i<array1.length;i++)
- for(int j=0;j<array2.length;j++)
- Check for condition
- if(array[i]==(array2[j]))
- print array[i];
- else print there is no common element
- end
import java.util.*;
import java.util.Arrays;
public class CommomElement
{
public static void main(String args[])
{
int array1[]={10,20,30,40,50};
int array2[]={15,25,40,35,60};
System.out.println("Array1:"+Arrays.toString(array1));
System.out.println("Array2:"+Arrays.toString(array2));
for(int i=0;i<array1.length;i++)
{
for(int j=0;j<array2.length;j++)
{
if(array1[i]==array2[j])
{
System.out.println("Common Element between two array:"+(array1[i]));
}
else("No common Elements");
}
}
}
}
Program-to-find-common-elements-between-two-arrays
Example2: Taking User-Defined input
Algorithm/Program Explanation
- start
- define variable int size.
- take input for size using Scanner class.
- define and initialize two array
- int array1[]=new int[size];
- int array2[]=new int[size];
- define two for loop to take input for array1 & array Respectively.
- define 2 for loops
- for(int i=0;i<array1.length;i++)
- for(int j=0;j<array2.length;j++)
- Check for condition
- if(array[i]==(array2[j]))
- print array[i];
- else print there is no common element
- end
//Program
import java.util.Arrays;
import java.util.Scanner;
public class CommonElement
{
public static void main(String args[])
{ int size;
System.out.println("Enter Size of Both Array");
Scanner sc=new Scanner(System.in);
size=sc.nextInt();
int array1[]=new int[size];
int array2[]=new int[size];
System.out.println("Enter Element of Array1");
for(int i=0;i<size;i++)
{
array1[i]=sc.nextInt();
}
System.out.println("Enter Element of Array2");
for(int j=0;j<size;j++)
{
array2[j]=sc.nextInt();
}
System.out.println("Array1:"+Arrays.toString(array1));
System.out.println("Array2:"+Arrays.toString(array2));
for(int i=0;i<array1.length;i++)
{
for(int j=0;j<array2.length;j++)
{
if(array1[i]==array2[j])
{
System.out.println("Common Element between two array:"+(array1[i]));
}
else("No common Elements");
}
}
}
}
Enter Size of Both Array
5
Enter Element of Array1
10 20 30 40 50
Enter Element of Array2
15 25 40 35 60
Array1:[10, 20, 30, 40, 50]
Array2:[15, 25, 40, 35, 60]
Common Element between two array:40
Question Asked in this Topic:
- Write a Java program to Display Common Elements between two integer arrays.
- Given are the two integer arrays write a logic to find common elements between them.
- Take two user-defined arrays and compare if there exists a common element between them, if yes then print a common element, Else print no common element.