Write a Java Program to sort Array Elements in Ascending order
In this we will be taking user defined array with the help of Scanner class. After successful input all elements in array will apply simple sorting logic on it,i.e. comparing every element with each elements in an array.
Logic:
Java program to sort array elements in ascending order
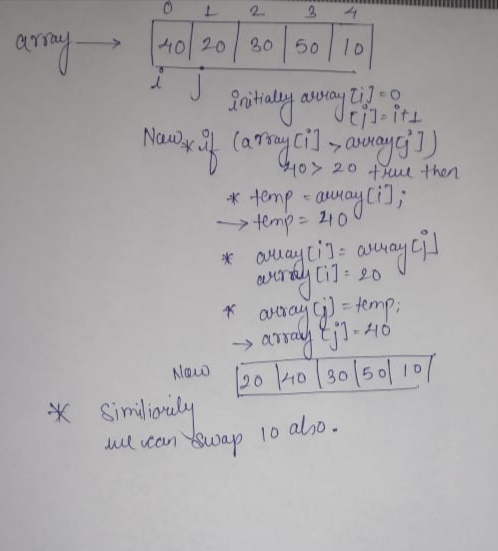
/*
java program to sort array elements in ascending order */
import java.util.*;
import java.util.Scanner;
public class ArrayAscending{
public static void main(String args[]){
int size,temp;
Scanner sc=new Scanner(System.in);
System.out.println("Enter the Size of Array:");
size=sc.nextInt();
int array[]=new int[size];
System.out.println("Enter elements in array:");
for(int i=0;i<size;i++)
{
array[i]=sc.nextInt();
}sc.close();
for(int i=0;i<size;i++)
{
for(int j=i+1;j<size;j++)
{
if(array[i]>array[j]){
temp=array[i];
array[i]=array[j];
array[j]=temp;
}
}
}
System.out.println("Arrays Elements in Ascending order are:");
for(int i=0;i<size-1;i++)
{
System.out.println(array[i]+"|");
}
System.out.println(array[size-1]);
}
}
Output:
Enter the Size of Array:
5
Enter elements in array:
90 55 10 35 25
Arrays Elements in Ascending order are:
10|25|35|55|90
There is one another method to sort array elements in ascending order:
sort() is a method in java.util.Arrays class.
Syntax: sort(int array[], int from_index, int to_index)
Where: array[]: Array to be sorted.
from_index: Index of the element from where you want to sort array.
to_index: Index of the last element till you want to sort array.
Java Program to sort array element using Arrays.sort() method
import java.util.Arrays;
public class arraysort
{
public static void main(String args[])
{
int array[]={90,55,10,35,5,25};
Arrays.sort(array);
System.out.print("Sorted array:"+ Arrays.toString(array));
}
}
Output:
Sorted array: 10 25 35 55 90
Java Program to sort Array Elements in Descending order
/*
java program to sort array elements in descending order */
import java.util.*;
import java.util.Scanner;
public class ArrayAscending{
public static void main(String args[]){
int size,temp;
Scanner sc=new Scanner(System.in);
System.out.println("Enter the Size of Array:");
size=sc.nextInt();
int array[]=new int[size];
System.out.println("Enter elements in array:");
for(int i=0;i<size;i++)
{
array[i]=sc.nextInt();
}sc.close();
for(int i=0;i<size;i++)
{
for(int j=i+1;j<size;j++)
{
if(array[i]>array[j])//descending logic{
temp=array[i];
array[i]=array[j];
array[j]=temp;
}
}
}
System.out.println("Arrays Elements in descending order are:");
for(int i=0;i<size-1;i++)
{
System.out.print(array[i]+"|");
}
System.out.print(array[size-1]);
}
}
Output:
Enter the Size of Array:
5
Enter elements in array:
90 55 10 35 5 25
Arrays Elements in descending order are:
90|55|35|55|10|
Important Question can be asked in this topic
- Write a program to sort array in ascending order?
- Writ a program to sort array elements using Arrays.sort() method?
- Demonstrate the use of Arrays.sort method?
- Write a java program to sort array in descending order?