Java Program to Multiply two or more matrices is one of the most frequently asked interview and coding question,therefore it is necessary to understand logic to implement this program.Don’t worry I will make you understand it. In this topic we will see two examples.In Example-1 we will take Static Inputs(Pre-defined Inputs). And in Example-2 we will take User defined Inputs.
How Matrices are Multiplied
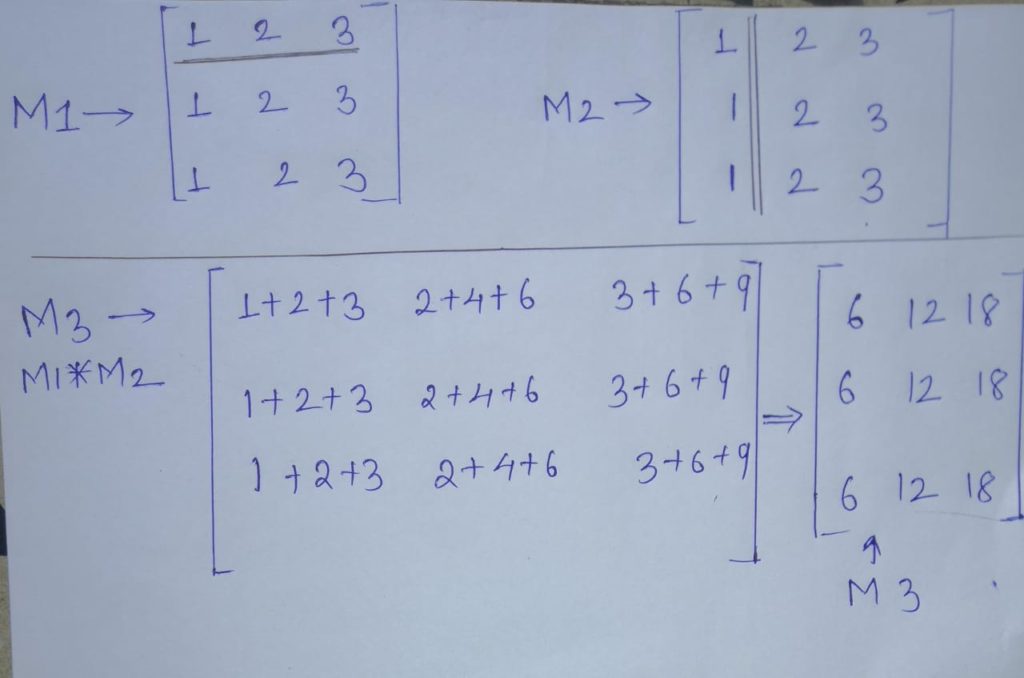
Lets take 1st row of matrix1 and all three columns of matrix3 [1 2 3] X | 1 2 3 | | 1 2 3 | | 1 2 3 | [ 1*1+2*1+3*1 1*2+2*2+3*2 1*3+2*3+3*3] Procedure:- 1.Here 1st element of first row of matrix1 is multiplied with 1st element of first columns of matrix2 i.e 1*1. 2.Then 2nd element of first row of matrix1 is multiplied with 2nd element of first columns of matrix2 i.e 2*1 3.Then 3rd element of first row of matrix1 is multiplied with 3rd element of first columns of matrix2 i.e 3*1 4.Now add all the result of multiplication i.e. 1+2+3=6 5. '6' is the first entry for matrix3 Note:In this way fill more 8 entries in matrix3
Algorithm/Program Explanation
- Start
- define and initialize three 2d array
- int matrix1[][]={{1,2,3}, {1,2,3}, {1,2,3}};
- int matrix2[][]={{1,2,3}, {1,2,3}, {1,2,3}};
- int matrix3[][]=new int[3][3];// enter data accordingly
- Define Three for loop
- for(int i=0;i<3;i++).
- for(int j=0;j<3;j++)
- for(int m=0;m<3;m++)
- Condition:
- matrix3[i][j]+=matrix1[i][m]*matrix2[m][j];
- Print matrix3
- end
Example-1 Taking Static Inputs:
/*
Java Program for Matrix Multiplication */
import java.util.*;
public class MatrixMultiplication {
public static void main(String args[]){
int matrix1[][]={{1,2,3}, {1,2,3}, {1,2,3}};
int matrix2[][]={{1,2,3}, {1,2,3}, {1,2,3}};
int matrix3[][]=new int[3][3];
System.out.println("Matrix After Multiplication:");
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
for(int m=0;m<3;m++){
matrix3[i][j]+=matrix1[i][m]*matrix2[m][j];
}System.out.print(matrix3[i][j]+ " ");
} System.out.println();
}
}}
Output:
Matrix After Multiplication:
6 12 18
6 12 18
6 12 18
Example-2 Taking User Defined Inputs
/*
Java Program for Matrix Multiplication */
import java.util.*;
import java.util.Scanner;
public class MatrixMultiplication {
public static void main(String args[]){
Scanner sc=new Scanner(System.in);
int matrix1[][]= new int [3][3];
int matrix2[][]= new int [3][3];
int matrix3[][]=new int[3][3];
System.out.println("Enter The Elements of Matrix-1");
for(int i=0;i<3;i++)
{ for(int j=0;j<3;j++){
matrix1[i][j]=sc.nextInt();
}
}
System.out.println("Enter The Elements of Matrix-2");
for(int i=0;i<3;i++)
{ for(int j=0;j<3;j++){
matrix2[i][j]=sc.nextInt();
}
}
System.out.println("Matrix After Multiplication:");
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
for(int m=0;m<3;m++){
matrix3[i][j]+=matrix1[i][m]*matrix2[m][j];
}System.out.print(matrix3[i][j]+ " ");
} System.out.println();
}
}}
Output:
Enter The Elements of Matrix-1
1 2 3
1 2 3
1 2 3
Enter The Elements of Matrix-2
1 2 3
1 2 3
1 2 3
Matrix After Multiplication:
6 12 18
6 12 18
6 12 18
Question Asked in This Topics
- Write a Java Program to Multiply two Matrices?
- Derive a Logic for Matrix Multiplication
- Given are the 2 matrices implement java logic multiply them?
- Take user defined matrices and code a logic to multiply them?
Related Topics:
- Arithmetic Operations Java Program
- Java program to perform Logical and Relational operations
- Java program to Implement Mathematical functions defined in Java Math class
- Java basic Programs with Explanation
- Java Program to find Min,Max Element in an Array
- Java Program to sort Array Elements in ascending and descending order
- Java Program to display Array item at Even & Odd position
- Program to Calculate Sum and Average of Array elements
- Java Program to find Index of specific Element in an array
- Program to find common elements between two arrays
- Java Program to Add/Insert specific Element to an Array
- Java Program to delete specific element from an Array
- Program to calculate frequency of each element in Array
- Java Program to find Even and Odd Numbers in the arrays
- Java Program to search Given Number in an Array
- Java Program to Add or Sub Two Matrices
- Java Program to Multiply two or More Matrices
- Java Program to Print Array in Reverse Order
- Java Program To Transpose Given Matrix
- Java Programs List- Java Programs Examples With Output