There occurs a situation where we wants to add a particular element to an array,So using this program we can serve this purpose.We will take two example for this program.In Example-1 we will take Static input(pre-defined input). And in Example-2 we will take inputs from users.
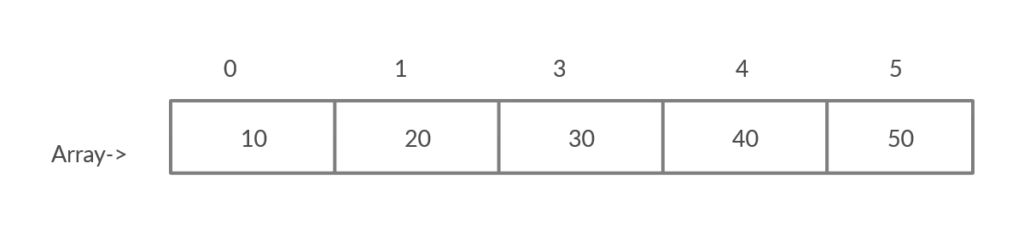
Above Example depicts an array integer of size 5,Suppose if we want to add 60 at position 1 so our program should add 60 at position 1 and shift the position of other elements by 1.
Example1: Using Static Input
Algorithm/Program Explanation
- Start.
- define and initialize integer array.
- int array[]={data};
- define two variable-
- int index=value; // Index location where you want to add element.
- int newnum=value; //New Number you want to add.
- print original array before any operation for better understanding.
- define for loop
- for(int i=size-1;i>index;i–)
- array[i+1]=array[i];
- array[index-1]=newnum;
- print new array;
/*java program to insert element at specific location
*/
import java.util.Arrays;
import java.util.Scanner;
public class Insertelement{
public static void main(String[] args) {
int size ,index=2,newnum=60;
System.out.println("Enter Size of array:");
Scanner sc=new Scanner(System.in);
size=sc.nextInt();
int[] array = new int[size+1];
System.out.println("Enter All ELements in Array:");
for(int i=0;i<size;i++){
array[i]=sc.nextInt();
}
System.out.println("Original Array" + Arrays.toString(array));
int i;
for (i = size - 1; i > index; i--) {
array[i+1] = array[i];
}
array[index-1] = newnum;
System.out.println("New Array:" + Arrays.toString(array));
}
}
Enter Size of array:
5
Enter All ELements in Array:
10 20 30 40 50
Original Array[10, 20, 30, 40, 50, 0]
New Array:[10, 60, 30, 40, 40, 50]
Example 2: Taking User Defined Inputs
/*java program to insert element at specific location
*/
import java.util.Arrays;
import java.util.Scanner;
public class Insertelement{
public static void main(String[] args) {
int size ,index,newnum;
System.out.println("Enter Size of array:");
Scanner sc=new Scanner(System.in);
size=sc.nextInt();
int[] array = new int[size+1];
System.out.println("Enter All ELements in Array:");
for(int i=0;i<size;i++){
array[i]=sc.nextInt();
}
System.out.println("Enter the Index where you want to Add/Insert Element");
index=sc.nextInt();
System.out.println("Enter the Value of New Number you want to Add/Insert Element");
newnum=sc.nextInt();
System.out.println("Original Array" + Arrays.toString(array));
int i;
for (i = size - 1; i > index; i--) {
array[i+1] = array[i];
}
array[index-1] = newnum;
System.out.println("New Array:" + Arrays.toString(array));
}
}
Output:
Enter Size of array:
5
Enter All ELements in Array:
10 20 30 40 50
Enter the Index where you want to Add/Insert Element
2
Enter the Value of New Number you want to Add/Insert Element
60
Original Array[10, 20, 30, 40, 50, 0]
New Array:[10, 60, 30, 40, 40, 50]
Question asked in this Topic
- Write a program to insert element at specific location?
- Write a logic to add element in an array?
- Define user define array add element to it and also add new element to it?